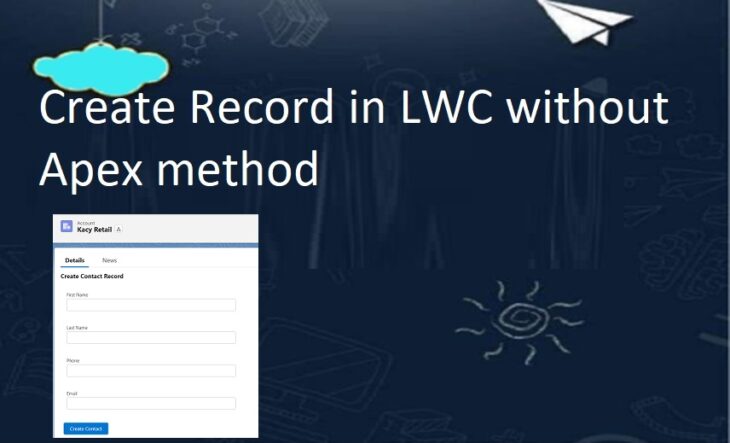
CreateRecord by LWC without Apex method in Salesforce
This post will help to implement the basic functionality to create records in LWC using createRecord of uiRecordApi module without Apex code. salesforce Library
createRecord
uses this User Interface API resource, but doesn’t support all its parameters
The createRecord() with objRecordInput as a parameter. This method returns a Promise. A Promise can be either in pending, fulfilled, or rejected state. A pending promise can either be fulfilled with a value or rejected with an error. Hence we need to handle only a fulfilled and rejected state. When the Promise returned by createRecord() is successful or fulfilled, then() will be executed. If it returns an error, then the catch() function is executed.
So I have created a LWC component that’s will create a contact record with help of input fields without apex code:
CreateContactRecord.html
<template>
<lightning-card title="Create Contact Record">
<lightning-layout>
<lightning-layout-item size="6">
<!-- Displaying fields to get information. -->
<lightning-input class="slds-p-around_medium" label="First Name" name="FirstName"
onchange={fNameChangedHandler}></lightning-input>
<lightning-input class="slds-p-around_medium" label="Last Name" name="LastName"
onchange={lNameChangedHandler}></lightning-input>
<lightning-input class="slds-p-around_medium" label="Phone" type="phone" name="Email"
onchange={phoneChangedHandler}></lightning-input>
<lightning-input class="slds-p-around_medium" label="Email" type="Email" name="Email"
onchange={emailChangedHandler}></lightning-input>
<br/>
<lightning-button class="slds-m-left_x-small" label="Create Contact" variant="brand"
onclick={createContact}></lightning-button>
</lightning-layout-item>
</lightning-layout>
</lightning-card>
</template>
CreateContactRecord.js
import { LightningElement,api } from 'lwc';
import { createRecord } from 'lightning/uiRecordApi';
export default class CreateContactRecord extends LightningElement {
@api recordId;
firstNameField;
lastNameField;
emailField;
phoneField;
// Change Handlers.
fNameChangedHandler(event){
this.firstNameField = event.target.value;
}
lNameChangedHandler(event){
this.lastNameField = event.target.value;
}
emailChangedHandler(event){
this.emailField = event.target.value;
}
phoneChangedHandler(event){
this.phoneField = event.target.value;
}
createContact(){
// Creating mapping of fields of Contact with values
var fields = {'FirstName' : this.firstNameField,
'LastName' : this.lastNameField,
'Email' : this.emailField,
'Phone' : this.phoneField ,
'AccountId' : this.recordId
};
// Record details to pass to create method with api name of Object.
var objRecordInput = {'apiName' : 'Contact', fields};
createRecord(objRecordInput).then(response => {
alert('Contact Record Created With Id: ' +response.id);
}).catch(error => {
alert('Error: ' +JSON.stringify(error));
});
}
}
CreateContactRecord.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>56.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>