Lightning Interview Questions
<<<<<<LWC Interview Questions>>>>>>
1. What is LWC(Lightning Web Components)?
As of now, we have built a lightning component using the “Aura Components model”. we can also build a lightning component using the “Lightning Web Components model”. Lightning Web Components are custom HTML elements build using the HTML Elements and modern Javascript. We can build components using any of the models and can place these components on the same lightning page.
For developing LWC we require “Salesforce Extensions for Visual Studio Code” and for deploying LWC from an org we require “Salesforce CLI”.
Aura Components make use of own custom component model, custom templates, custom components, etc while LWC is built on web standards and it makes use of web components, templates, custom elements which we have in web standards. Both the Aura component, LWC make use of Security, LDS and Base lightning components.
Important points to note:
1) Aura component and LWC can exist on the same lightning page.
2) Aura component can include LWC
2. What is Scratch org?
Scratch org is a disposable Salesforce org used for development and testing.
Scratch org can be created for a maximum of 30 days after which scratch org gets deactivated. The default duration for Scratch org is 7 days.
3. Explain the Lightning Web Component Bundle?
LWC bundle contains an HTML file, a JavaScript file, and a metadata configuration file
and these files are created once we create a Lightning web component.
We can also create a .css file for styling purpose and We can also create SVG file for the purpose of displaying icon.
4. How to render the HTML file conditionally?
<template if:true={areDetailsVisible}>
</template>
<template if:false={areDetailsVisible}>
</template>
5. How to iterate over an array in HTML file?
We can make use of for:each directive and iterator directive.
for:each:
for:each directive is used to render an array. To render an array add the for:each directive to a nested template tag, for:item is used to access the current item, for:index is used to access the current index.
<template>
<!--for:each-->
<b>for:each directive</b>
<template for:each={students} for:item="item" for:index="index">
<p key={item.id}>
{item.Name}
{item.Branch}
</p>
</template>
Iterator:
If you have the requirement to access the first and the last element in the list use the iterator directive.
<template iterator:it={students}>
<li key={it.value.id}>
{it.value.id}
{it.value.Name}
{it.value.Branch}
</li>
<!--To access the first and the last element use {it.first} and {it.last}-->
</template>
6. What are the types of decorators in lightning web components?
1) @api:- To expose a public property, decorate a field with @api
. Public properties define the API for a component.
2) @track:- It is used to make variable private but reactive. Tracked properties are also called private reactive properties. If a field’s value changes, and the field is used in a template or in a getter of a property that’s used in a template, the component rerenders and displays the new value.
If a field is assigned an object or an array, the framework observes some changes to the internals of the object or array, such as when you assign a new value.
To use @track we have to import it first from lwc.
Import @track decorator from lwc
<template>
<div class="slds-m-around_medium">
<p>Hello, {greeting}!</p>
<lightning-input label="Name" value={greeting} onchange={changeHandler}></lightning-input>
</div>
</template>
import { LightningElement, track} from 'lwc';
export default class ChildComp extends LightningElement {
@track greeting = 'Hello World';
changeHandler(event) {
this.greeting = event.target.value;
}
}
Note: You can use “greeting” variable without @track but is always recommended to use @track in case you are showing variable in html as it make it reactive so whenever it changes in js the changes will also be visible in html.
Observe an Object’s Properties or an Array’s Elements
To understand, let’s declare the fullName
field, which contains an object with two properties, firstName
and lastName
.
fullName = { firstName : '', lastName : '' };
The framework observes changes that assign a new value to fullName
. This code assigns a new value to the fullName
field, so the component rerenders.
// Component rerenders.
this.fullName = { firstName : 'Mukesh', lastName : 'Gupta' };
As below, if we assign a new value to one of the object’s properties, the component doesn’t rerender because the properties aren’t observed.
// Component doesn't rerender.
this.fullName.firstName = 'Kumar';
The framework observes changes that assign a new value to the fullName
field. This code doesn’t do that, instead it assigns a new value to the firstName
property of the fullName
object.
To tell the framework to observe changes to the object’s properties, decorate the fullName
field with @track
. Now if we change either property, the component rerenders.
// Component rerenders.
@track fullName = { firstName : '', lastName : '' };
this.fullName.firstName = 'Mukesh';
3) @wire:- To read Salesforce data, Lightning web components use a reactive wire service. When the wire service provisions data, the component rerenders. Components use @wire in their JavaScript class to specify a wire adapter or an Apex method.
We need to import the@salesforce/apex
scoped module into JavaScript controller class.
Here is list of important point of importing apex method:
- apexMethodName : An imported symbol that identifies the Apex method.
- apexMethodReference : The name of the Apex method to import.
- Classname : The name of the Apex class.
- Namespace—The namespace of the Salesforce organization. Specify a namespace unless the organization uses the default namespace (c), in which case don’t specify it.
Example:- How to access apex class method from @wire
public with sharing class AccountHelper {
@AuraEnabled(cacheable=true)
public static List<Account> getAccountList() {
return [SELECT Id, Name, Type, Rating, Phone FROM Account];
}
}
Note: ApexMethod should be annotated with @AuraEnabled
and if you use cacheable=true
you can’t mutate data in your apex method.
How to use @wire method in LWC component:-
import { LightningElement, wire } from 'lwc';
import getAccountList from '@salesforce/apex/AccountHelper.getAccountList';
export default class ChildComp extends LightningElement {
@wire(getAccountList) accounts;
}
7. How many scratch orgs we can create daily, and how many can be active at a given point?
Our “Dev Hub” org edition determines how many scratch orgs we can create daily, and how many can be active at a given point.
8. How many files are created by default when we create a Lightning Web Component?
Three files are created as below.
HTML file.
JavaScript file.
Meta configuration file.
9. What is the purpose of .css file and svg file in Lightning Web Component bundle?
.css file is use for styling purpose and svg file is use for purpose of displaying icon.
10. What is the purpose of HTML file in Lightning Web Component bundle?
- The HTML file is enclosed within the <template> tags.
- It contains the component HTML.
<template>
<h1> Some Text </h1>
</template>
11. How we can build Lightning web components?
It is always recommended to use Salesforce DX tools for developing Lightning web components however we can still use code editor to develop Lightning web components.
Note: we cannot build LWC in the Developer Console.
12. How can we use Lightning web component with unsupported tool?
To use a Lightning web component with an unsupported experience or tool we can wrap it in an Aura component.
13. What is the purpose of Javascript file in Lightning Web Component bundle?
- The Javascript file defines the behaviour of HTML element present in HTML file.
- JavaScript files in Lightning web components are ES6 modules. By default, everything declared in a module is local—it’s scoped to the module.
- If we want to import a class, function, or variable declared in a module we use the import statement.
- If we want to allow other code to use a class, function, or variable declared in a module we use the export statement.
- The core module in Lightning Web Components is lwc. The import statement imports LightningElement from the lwc module.
import { LightningElement } from ‘lwc’;
Here, LightningElement is a custom wrapper of the standard HTML element.
Extend LightningElement to create a JavaScript class for a Lightning web component. You can’t extend any other class to create a Lightning web component.
export default class MyComponent extends LightningElement {
// Code
}
14. What is Meta configuration file in Lightning Web Component?
The Meta configuration file defines the metadata values for the components.
If we don’t include a configuration file for our component, we get an error similar to the following when we push your changes.
Cannot find Lightning Component Bundle <component_name>
15. Is it possible to use multiple html files in a Lightning Web Component?
When working with lightning web components there may be times when you want to render a component with different looks that are dependent on the data passed in. Instead of mixing all the different styles in one file, we can have multiple HTML templates in the same component and render them conditionally.
Firstly, we create multiple HTML files in one of our LWC component bundles. Then we import the HTML files in our javascript file.
We can use an expression evaluation inside the render() method to return the correct HTML file. We can also have a method that will toggle the method that is rendered.
import {LightningElement, api} from 'lwc';
import optionA from './optionA.html';
import optionB from './optionB.html';
export default class MultipleHtmlTemplatesExample extends LightningElement {
displayOptionA = false;
render(){
//If displayOptionA is true return optionA template
return this.displayOptionA ? optionA : optionB;
}
toggleOptions(){
this.displayOptionA = !this.displayOptionA;
}
}
16. How many style sheet can a component have?
Each component can have only one style sheet.
17. Can components share the style sheet?
No.
18. Does the style of the component get lost or override when we use the component with its own style sheet in different context?
When we define a style sheet for a component in a component folder it is limited to that component only and this avoid loosing the style sheet of the component when we reuse this component in different contexts. This also avoid component style sheet getting override in other part of the page.
19. How to share CSS style sheet in Lightning Web Component?
1) If we want to share CSS style than we will need to create a module that contains only a CSS file and for this we will need to create a Lightning Web Component with only single CSS file as shown below.
2) After we create a module we can import the module into CSS file of any Lightning Web Component as shown below.
@import ‘namespace/nameOfModule’;
20. What is used to display toast notifications in Lightning Web Component?
Toast notification pops up to alert users of a success, error, or warning. We need to import ShowToastEvent from the lightning/platformShowToastEvent module to display a toast notification in Lightning Experience or Experience Builder sites.
21. List the callback methods that handles life cycle of LWC?
A lifecycle hook is a callback method triggered at a specific phase of a component instance’s lifecycle.
Lightning web components have a lifecycle managed by the framework. The framework creates components, inserts them into the DOM, renders them, and removes them from the DOM. It also monitors components for property changes. Generally, components don’t need to call these lifecycle hooks, but it is possible.
constructor():-
Called when the component is created. This hook flows from parent to child. You can’t access child elements in the component body because they don’t exist yet. Properties are not passed yet, either.
connectedCallback():-
Called when the element is inserted into a document. This hook flows from parent to child. You can’t access child elements in the component body because they don’t exist yet.
disconnectedCallback():-
It gets invoked automatically when the corresponding web component gets removed from DOM. If we need to perform any logic when the component is removed from DOM, that logic can be added in disconnectedCallback(). This hook flows from Parent component to child. We use disconnectedCallback() to purging caches or removing event listeners that are created on connectedCallback().
render():-
Call this method to update the UI. It may be called before or after connectedCallback().
It’s rare to call render() in a component. The main use case is to conditionally render a template. Define business logic to decide which template (HTML file) to use. The method must return a valid HTML template.
For example, imagine that you have a component that can be rendered in two different ways but you don’t want to mix the HTML in one file. Create multiple HTML files in the component bundle. Import them both and add a condition in the render() method to return the correct template depending on the component’s state.
renderedCallback():-
Called after every render of the component. This lifecycle hook is specific to Lightning Web Components, it isn’t from the HTML custom elements specification. This hook flows from child to parent.
A component is rerendered when the value of property changes and that property is used either directly in a component template or indirectly in the getter of a property that is used in a template.
errorCallback(error, stack):-
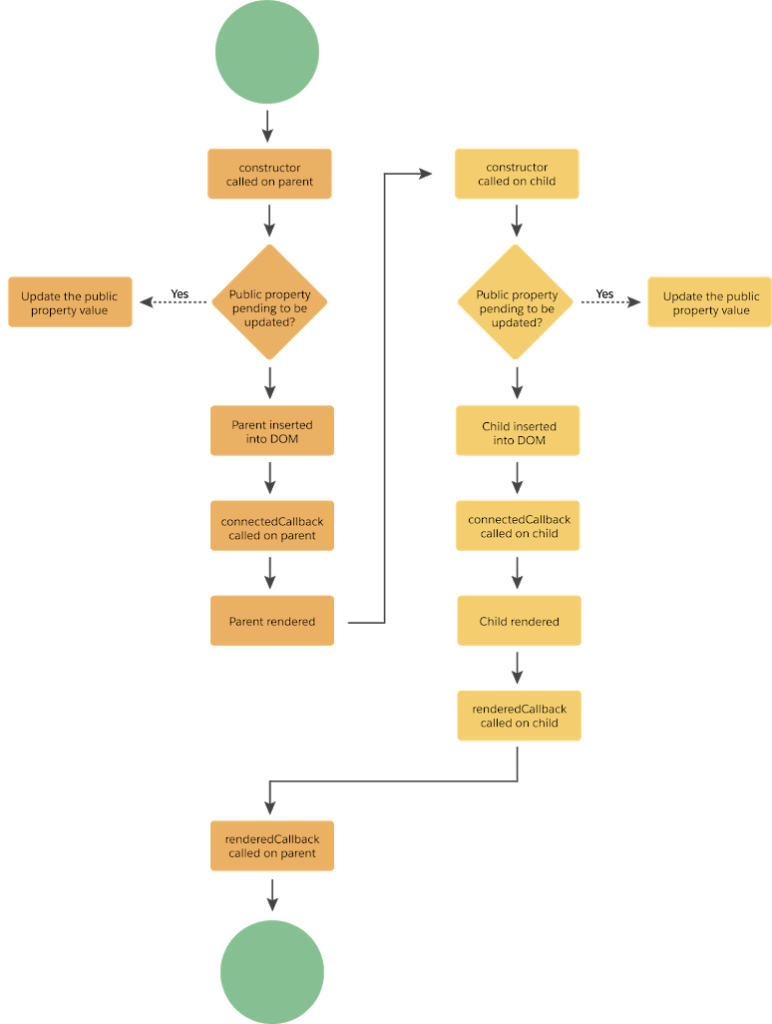
22. How to pass data from child component to parent component in lightning web component?
To pass data from child component to parent component we can trigger event from child LWC and handled it parent LWC as shown in below example.
23. Can we call the @AuraEnabled function in the apex class using wire ?
Yes, @AuraEnabled is mandatory when we try to fetch apex class method. Function also needs to have cacheable = true annotation like @AuraEnabled(cacheable = true)
24. Will the below code deploy?
@wire(getAccount,{accId : this.accId})
getAccList(error,data){}
NO, because variable in wire should always use $ along with variable. So need to use below code:-
@wire(getAccount,{accId: '$accId'})
25. What do you mean by cacheable = true annotations ?
First of all when you mark function as cacheable = true it can only retrieve data i.e. it cant have any DML.
It is used to improve component performance by quickly showing cached data from client side storage without waiting for server trip.
Remember to refresh the stale data provisioned by Apex we have to use refreshApex as LDS doesn’t manage data coming from Apex ( In case of wired service)
26. Why do we use $ when passing property in wire function, what does it mean?
$ prefix tells the wire service to treat values passed as a property of the class and evaluate it as this.propertyName and the property is reactive. If the property’s value changes, new data is provisioned and the component renders.
27. Is wire method called multiple times during lifecycle of component ?
Yes
27. What are lifecycle hooks in LWC ?
A lifecycle hook is a callback method triggered at a specific phase of a component instance’s lifecycle.
There are following hooks supported in LWC :
Constructor : Called when the component is created.
Connectedcallback : Called when the element is inserted into a document. This hook flows from parent to child.
RenderedCallback : Called after every render of the component. This lifecycle hook is specific to Lightning Web Components, it isn’t from the HTML custom elements specification. This hook flows from child to parent. Ie its not part of HTMLElement rather defined in LightningElement.
Disconnectedcallback : Called when the element is removed from a document. This hook flows from parent to child.
Errorcallback : Called when a descendant component throws an error. The error argument is a JavaScript native error object, and the stack argument is a string. This lifecycle hook is specific to Lightning Web Components, it isn’t from the HTML custom elements specification
28. If I want to refresh the wired data in the below function, can I call refreshApex(this.accounts) ?
@wire(getAccountList)
wiredAccounts(error,data) {
this.wiredAccountsResult = result;
if (data) {
this.accounts = data;
this.error = undefined;
} else if (result.error) {
this.error = error;
this.accounts = undefined;
}
}
No we cant call refreshApex(this.accounts) rather refreshApex is called on whole result provisioned from the wire service not just the data part, we will have to rewrite it as below :
@wire(getAccountList)
wiredAccounts(result) {
this.wiredAccountsResult = result;
if (result.data) {
this.accounts = result.data;
this.error = undefined;
} else if (result.error) {
this.error = result.error;
this.accounts = undefined;
}
}
Now will work as refreshApex(this.wiredAccountsResult)
29. Can we call a wire function inside a javascript function like below :
searchAccounts(event){
@wire(getAccount,{accId: '$accId'})
getAccList(data,error){
}
}
We can’t call it like this , code will not deploy. You will receive error as leading decorators must be attached to a class which means decorators like wire can be directly under class only not inside any other function.
Similarly if you try to define variable with @track or api decorator inside a function it will fail.
30. When is the wire method/property called in the lifecycle of a component ?
Wired service is called right after component is created ie after constructor and is again called when parameter that you are passing is made available.
31. What is the difference in below two codes , will they both compile ? Give same results ?
CODE 1:
@wire(getAccountList)
wiredAccounts(data,error) {
if (data) {
console.log('Success ENTER');
} else if (error) {
console.log('Failure ENTER');
}
}
CODE 2:
@wire(getAccountList, {})
wiredAccounts(data,error) {
if (data) {
} else if (error) {
}
}
Both will compile because they are same.
32. Is it mandatory to use data,error only in wired method, can I use some other variable like below
@wire(getAccountList)
wiredAccounts(myData,myError) {
if (myData) {
console.log('Success ENTER');
} else if (myError) {
console.log('Failure ENTER');
}
}
We cant use any other variable name other than data, error they are hardcoded in wire service. Code will successfully deploy but wire service wont be able to fetch any data.
33. Are quick actions supported for LWC components ?
Quick actions are supported by LWC in Summer 21 or later orgs. LWC quick actions are only supported on record pages.
There are two types of LWC quick actions: screen quick actions and headless quick actions.
- A screen quick action displays a component in a modal window
- A headless quick action executes custom code you provide in an
@api invoke()
method
This configuration file defines a screen action:-
<?xml version="1.0" encoding="UTF-8" ?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordAction</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>ScreenAction</actionType>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
This configuration file defines a headless action:-
<?xml version="1.0" encoding="UTF-8" ?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordAction</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordAction">
<actionType>Action</actionType>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
34. What is the difference between event.StopPropogation() and Event.preventDefault()?
stopPropagation() event method: This method is used to prevent the parent element from accessing the event.
For Example:-
<body>
<div class="first" onclick="functionFirst()">
<button onclick="functionSecond()">
Button
</button>
</div>
<script>
function functionFirst() {
alert("div hello"); // Second number execute
}
function functionSecond() {
alert("button hello"); //First number execute
}
</script>
</body>
</html>
OUT PUT:-
button hello
div hello
If we don’t want to fire Parent button then, We can solve this problem by using the stopPropagation() method because this will prevent the parent from accessing the event.
Now, in this case, we have added an event.stopPropagation() method, then the only function of the button element will be executed.
<body>
<div class="first" onclick="functionFirst()">
<button onclick="functionSecond()">
Button
</button>
</div>
<script>
function functionFirst() {
alert("div hello"); // Will Not execute
}
function functionSecond() {
event.stopPropagation();
alert("button hello"); // Execute this only
}
</script>
</body>
preventDefault() Method: It is a method present in the event interface. This method prevents the browser from executing the default behavior of the selected element. This method can cancel the event only if the event is cancelable.
Example 1: Preventing a link from following the URL so that the browser can not go to another page.
<body>
<a id="first" href="https://uniquesymbol.com/">
UniqueSymbol
</a>
<script>
$("#first").click(function () {
event.preventDefault(); // above url will not open
alert("Event prevented, Can't go there.");
});
</script>
</body>
35. If we add required attribute in lightning-input , will I get an error on leaving the field empty ?
No, we also need to add logic in backend javascript file to actually throw an error using checkValidity and reportValidity.
For example, you have the following form with several fields and a button. To display error messages on invalid fields, use the reportValidity()
method.
<template>
<lightning-input label="First name" placeholder="First name" required>
</lightning-input>
<lightning-input label="Last name" placeholder="Last name" required>
</lightning-input>
<lightning-button type="submit" label="Submit" onclick={handleClick}>
</lightning-button>
</template>
export default class InputHandler extends LightningElement {
value = 'initial value';
handleClick(evt) {
console.log('Current value of the input: ' + evt.target.value);
const allValid = [
...this.template.querySelectorAll('lightning-input'),
].reduce((validSoFar, inputCmp) => {
inputCmp.reportValidity();
return validSoFar && inputCmp.checkValidity();
}, true);
if (allValid) {
alert('All form entries look valid. Ready to submit!');
} else {
alert('Please update the invalid form entries and try again.');
}
}
}
Custom Validity Error Messages:-
<template>
<lightning-input class="inputCmp" label="Enter your name:">
</lightning-input>
<lightning-button label="Register" onclick={register}></lightning-button>
</template>
The register()
function compares the input entered by the user to a particular text string. If true, setCustomValidity()
sets the custom error message. The error message is displayed immediately using reportValidity()
.
import { LightningElement } from 'lwc';
export default class MyComponent extends LightningElement {
register(event) {
var inputCmp = this.template.querySelector('.inputCmp');
var value = inputCmp.value;
// is input valid text?
if (value === 'John Doe') {
inputCmp.setCustomValidity('John Doe is already registered');
} else {
inputCmp.setCustomValidity(''); // if there was a custom error before, reset it
}
inputCmp.reportValidity(); // Tells lightning-input to show the error right away without needing interaction
}
}
36. How can i reference record ID of page in my component which is fired from quick action.
In case of screen actions we will be able to get recordID by just defining recordID as public property in the component.
For headless action you have to define @api invoke method which is auto called and recordID will be set after this function is called.
In your Lightning web component, expose invoke()
as a public method. The invoke()
method executes every time the quick action is triggered.
export default class QuickActionComponent extends LightningElement {
@api recordId;
@api invoke() {
console.log( "Inside Invoke Method");
console.log( "Record Id is " + this.recordId );
}
}
37. What is a promise in async transactions? What are it different stages.
Promise is object returned in async transaction which notifies you about completion or failure of transaction
For example when you call apex imperatively it returns you a promise object on the basis of object returned execution either goes into (then) ie transaction was successful or (catch) which means transaction failed.
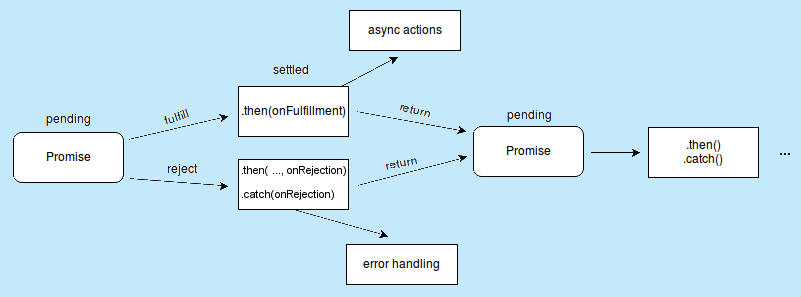
Stages of promises are :
Pending: Waiting for result.
Fulfilled: Promise results in success.
Rejected: Promise result in failure.
38. What is the difference between Promise and Promise.all ?
Promise takes in multiple promises in it and returns a single promise object.
Promise.all is used when I want to make sure once all promises are resolved then only execute then block.
var promise = new Promise(function(resolve, reject){ //do something });
Promise constructor takes only one argument, a callback function and the Callback function takes two arguments, resolve and reject. Perform operations inside the callback function and if everything went well then call resolve. If desired operations do not go well then call reject.
The Promise.all()
method takes an iterable of promises as an input, and returns a single Promise
that resolves to an array of the results of the input promises. This returned promise will fulfill when all of the input’s promises have fulfilled, or if the input iterable contains no promises. It rejects immediately upon any of the input promises rejecting or non-promises throwing an error, and will reject with this first rejection message / error.
const promise1 = Promise.resolve(3);
const promise2 = 42;
const promise3 = new Promise((resolve, reject) => {
setTimeout(resolve, 100, 'foo');
});
Promise.all([promise1, promise2, promise3]).then((values) => {
console.log(values);
});
// expected output: Array [3, 42, "foo"]
39. How do I retrieve elements on the basis of ID?
We should never use “id” in lwc as id that you will define in LWC may get transformed into something entirely different on rendering, rather use data-id in your tags to reference them.
40. Why do we need to extend LightningElement ?
LightningElement is custom wrapper on HTMLElement which actually contains all the lifecycle hooks methods over which Salesforce did some customization.
41. What are web components, Is LWC based on web components ?
It allows you to create and re-use custom components as html tags in a component with their functionality encapsulated from rest of your code.
Web components has 4 pillars
HTML Template : user defined templates which are rendered until called upon.
Custom Elements : With this we can embed as components merely as html tags in our template.
Shadow DOM : With this we can separate DOM of each custom element from each other to make sure nothing from any components leaks into each other.
HTML Imports : You can import other html documents in another html document for example we can import multiple templates into one component then use render function to display a template.
42. When we create new component why does it say export default ComponentName ?
Because of export default component allow to embed one component into another
43. How to send data from Parent to Child in LWC?
For parent to child communication you just need to expose your function or attribute as @api then in your parent component you can use querySelector to actually query the child component access exposed attribute or method.
We have 2 option for data communication from parent to child
Pass by component parameter:-
ParentComponent.html
<template>
<c-child-l-w-c datesend="parent value pass in child"></c-child-l-w-c>
</template>
ChildComponent.html
import { LightningElement, api } from 'lwc';
export default class ChildLWC extends LightningElement {
@api datasend;
}
Pass by component queryLocator:-
ParentComponent.html
import { LightningElement } from 'lwc';
export default class ParentComponent extends LightningElement {
const childComponentResponse = this.template.querySelector('c-child-component').callchildmethod('parent parameters');
}
ChildComponent.html
import { LightningElement, api } from 'lwc';
export default class ChildComponent extends LightningElement {
@api
callchildmethod(param1) {
// Do whatever...
return 'Done!';
}
}
44. What are callback functions in LWC ?
Callback functions are nothing but functions passed as parameter into other functions.
45. What does composed = true does in an event ?
please follow this url for better understanding:- https://developer.salesforce.com/docs/component-library/documentation/en/lwc/lwc.events_propagation
46. Does callback functions synchronous or asynchronous ?
Functions are neither async or sync in themselves rather depend on where are they being passed for example if a function in passed into reduce method is sync but if function is being passed into setTimeout function its async.
47. What is callback hell ?
callback hell occurs when there are many functions you want to call async and you end up puting them one side each another and as the code grows it becomes very difficult to read for example :
getData(function(x)
{
getMoreData(x, function(y)
{
getMoreData(y, function(z)
{ ... }
);
});
});
48. Can I use multiple decorators on one property ?
No we cant use multiple decorators on same property.
49. Can I deploy component with empty css file ?
No we can not do that !!
50. What is difference between var and let in JS ?
var is function scoped while let is block scoped
var allows you to redeclare same variable while let will throw an error
Var variables are hoisted that is you can access them even before they are declared in code its just they will return undefined value while with let it will throw an error.
51. Is there any difference in how we add LWC component inside another LWC component and inside AURA component ?
Difference is how they are added in the component.
LWC follows kebab case ie if you are adding any LWC component inside another component you will have to add in kebab case form while in AURA it will be added without kebab case for example :
We have component with name “childLWCComponent”
In LWC it will be added as <c-child-l-w-c-component/>
In Aura it will be added as <c:childLWCComponent/>
52. What is LMS ?
LMS is defined as the standard publish-subscribe library that enables communication with DOM across the components be it Visualforce Pages, Aura components, and Lightning Web Components (LWC) all can use it to publish message and listen to messages published by others.
53. Do we have application events in LWC?
We dont have application event as such in LWC like Aura rather we have LMS in LWC to communicate between components which are not part of same hierarchy
54. How to get current user ID in LWC without apex?
Yes, we can get current user ID without apex by simply importing
import Id from ‘@salesforce/user/Id’
55. How can we navigate user from LWC component to record detail page?
Can be done using NavigationMixin service
56. Do we have force:createRecord equivalent in LWC?
Using navigation mixin only you can also create new record where you define object , action as new and pass any default values you want to set.
// Navigate to New Account Page
navigateToNewAccountPage() {
this[NavigationMixin.Navigate]({
type: 'standard__objectPage',
attributes: {
objectApiName: 'Account',
actionName: 'new'
},
});
}
57. What is render() , is it part of lifecycle hook? Why do we use it ?
Render is not part of lifecycle hook its a protected function, we only use it if we have imported multiple templates in our component and we want to render particular template on meeting certain criteria.
58. What is String interpolation ?
In lightning AURA components, we have used concatenation lot of time to join multiple strings. But now we need to change something in the lightning web component.
when string literal is evaluated all the placeholders added into it are calculated at run time and replaced in string with values. Place holder can be anything a variable , expression even a function call. In javascript its performed using (backtick)
Example 1 :
const fName = 'Mukesh';
const lName= 'Gupta';
const fullName = ${fName}, ${lName}!`;
fullName // => ‘Mukesh, Gupta!’
59. What are template literals ? What is the use?
In javascript string interpolation is performed using template literals.
Template literals are created using (`) backtick character apart from string interpolation they also are used for adding multi-line strings without having to use “\n”
console.log('text line 1\n' +
'text line 2');
console.log(`text line 1
text line 2`);
//Both will result in same output
60. How can I evaluate expression in situation like <template if:true = {flag==true}
We cant add expressions directly in html file rather then what we can do is create getter which will evaluate value for us which we can use in html like below :
get isOk() { return this.flag = true; }
<template if:true ={isOk}
61. Why do we put constants outside of class in LWC?
We cant put constants inside a class or function in javascript its illegal for example below piece of code will throw you an error:-
export default class testClass extends LightningElement {
const testVar = 'testValue';
}
62. When do we face error of “Cant assign to Read only property” in LWC?
This error usually occurs when you are trying to make changes to a property which is marked as @api , ideally you should clone the value then make the changes to it.
@api someVar
code......
somemethod(event){
someVar.isSelected = true;
//error on above line - Cannot assign to read only property.
}
How to fix above error:-
@api someVar
.... some code
somemethod(event){
//clone @api variable using method we just created
let tmpObj = this.proxyToObj(someVar);
tmpObj.isSelected = true;
//now assign this tmp variable back to original
someVar = tmpObj;
}
63. Can i call function annotated with @AuraEnabled(cacheable= true) imperatively ?
Yes.
64. How to refresh cache when calling method imperatively
We have to use getRecordNotifyChange(RecordIds) which refreshes the LDS cache providing you the latest data this will work only if cacheable = true was there.
Otherwise we will have to call the function again from our js to get the latest data.
import { LightningElement, wire } from 'lwc';
import { getRecord, getRecordNotifyChange } from 'lightning/uiRecordApi';
import apexUpdateRecord from '@salesforce/apex/Controller.apexUpdateRecord';
export default class Example extends LightningElement {
@api recordId;
// Wire a record.
@wire(getRecord, { recordId: '$recordId', fields: ... })
record;
async handler() {
// Update the record via Apex.
await apexUpdateRecord(this.recordId);
// Notify LDS that you've changed the record outside its mechanisms.
getRecordNotifyChange([{recordId: this.recordId}]);
}
}
Benefits of using getRecordNotifyChange(recordIds)
- Update list of record Ids you’ve updated.
- It will only refresh records you ask for instead of complete page like force:refreshView event.
- Better performance.
- Faster as compare to the force:refreshView AURA event.
Note : Important thing is that, it will not bring new records to the local cache. For example if you’ve create a new record so using force:refreshView you may refresh the whole page and get the new records also in the related list but using getRecordNotifyChange() you can just update existing records in the related list.Hopefully it will have that update also in upcoming releases.
65. Can we do DML in method annotated with @AuraEnabled(cacheable= true)?
No we cant do DML inside any method annotated with cacheable = true , you will receive an error as DMLLimit Exception.
66. How to query all lightning-input , combobox, radio buttons using one querySelector or do I have to use multiple ?
We can query all of them using one query selector only no need to write multiple for each tag. We can pass all tags (,) separated to query all.
const allValid = […this.template.querySelectorAll(‘lightning-input,lightning-combobox,lightning-radio-group’)];
If you are wondering why are we using (…) spread operator before querySelectorAll its because querySelector returns you the node list and using spread operator we convert it to array of items otherwise we would not be able to use array functions like map or reduce.
67. What is reduce method in JS ?
reduce function is part of array prototype javascript object. It takes a function as input and applies that on the array and returns the output.
The reduce() method applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value.
Examples:-
var total = [0, 1, 2, 3].reduce(function(sum, value) {
return sum + value;
}, 0);
// total is 6
const students = [
{name: 'mike', id: 1, favsubject:'Science', rank: 1, percentage: 98},
{name: 'tom', id: 2, favsubject:'Maths', rank: 3, percentage: 96},
{name: 'nikole', id: 3, favsubject:'History', rank: 2, percentage: 97},
{name: 'willem', id: 4, favsubject:'Social', rank: 4, percentage: 95},
{name: 'wisten', id: 5, favsubject:'English', rank: 6, percentage: 79},
{name: 'tiff', id: 6, favsubject:'Science', rank: 5, percentage: 89},
];
let studentpercentages = students.map(eachstudent => eachstudent.percentage);
console.log(studentpercentages);
//reduce - as name suggests it reduces the array to single output
let averagepercentage = studentpercentages.reduce((eachstudent, nextstudent) => eachstudent + nextstudent);
console.log(averagepercentage/studentpercentages.length);
> Array [98, 96, 97, 95, 79, 89]
> 92.33333333333333
68. What is use of Object.assign(….)?
Object.assign – method copies all enumerable own properties from one or more source objects to a target object add new property including existing properties – merge
let newtiffrecord = Object.assign({fullName: 'tiff wols'}, tiffRecord);
console.log(newtiffrecord);
> Object { fullName: "tiff wols", name: "tiff", id: 1, favsubject: "Science", rank: 1, percentage: 98 }
69. What is arrow syntax =>?
=> expression simplifies the callback function notation. Lets take an example to understand it in detail.
let studentNames = students.map(eachstudent => {
return eachstudent.name;
});
console.log(studentNames);
With a regular function this
represents the object that calls the function:
/ Regular Function:
hello = function() {
document.getElementById("demo").innerHTML += this;
}
// The window object calls the function:
window.addEventListener("load", hello);
// A button object calls the function:
document.getElementById("btn").addEventListener("click", hello);
out put:-
onload:– [object Window]
button click:- [object HTMLButtonElement]
With an arrow function this
represents the owner of the function:
// Arrow Function:
hello = () => {
document.getElementById("demo").innerHTML += this;
}
// The window object calls the function:
window.addEventListener("load", hello);
// A button object calls the function:
document.getElementById("btn").addEventListener("click", hello);
output:-
onload:– [object Window]
button click:- [object Window]
Another Example:-
@wire(getAccounts)
wiredAccountRecord({data, error}) {
if(data){
this.settingsWrapper = ['A','B','C'];
data.forEach((elem,i) => {
/*setTimeout(function() {
// here this does not refer to Employee
console.log(this.settingsWrapper); // WILL GOT UNDEFINED this.settingsWrapper
}, 3000); */
setTimeout(() => {
console.log(this.settingsWrapper); // WILL GOT VALUE // A,B,C
},3000)
console.log('this.settingsWrapper ==>> '+this.settingsWrapper);
});
}else if(error){
}
}
70. What is difference between ‘ ==’ and ‘===’ ?
Both are used to compare two variables but == doesnt take data type into consideration and does type coercion ie interpreter tries to automatically convert data types to match the values while in === case if data type is not same it will always return false.
For example :
2=="2" will return True (Type coercion happens)
2==="2" will return False ( No Type coercion)
71. How to use static resources in LWC?
import myResource from '@salesforce/resourceUrl/namespace__resourceReference';
72. How to use external style sheet in LWC?
/* eslint-disable no-console */
import { api, LightningElement } from 'lwc';
import { loadStyle } from 'lightning/platformResourceLoader';
import styles from '@salesforce/resourceUrl/style';
export default class TestStyle extends LightningElement {
@api header
renderedCallback() {
console.log('styles')
console.log(styles)
loadStyle(this, styles)
.then(() => console.log('Files loaded.'))
.catch(error => console.log("Error " + error.body.message))
}
}
73. How to use refresh apex in LWC?
import { LightningElement, wire, track } from 'lwc';
import { deleteRecord } from 'lightning/uiRecordApi';
import { refreshApex } from '@salesforce/apex';
import getLatestAccounts from '@salesforce/apex/AccountController.getAccountList';
const COLS = [
{ label: 'Name', fieldName: 'Name', type: 'text' },
{ label: 'Stage', fieldName: 'Phone', type: 'text' },
{ label: 'Amount', fieldName: 'Industry', type: 'text' }
];
export default class LwcRefreshApex extends LightningElement {
cols = COLS;
@track selectedRecord;
@track accountList = [];
@track error;
@track wiredAccountList = [];
@wire(getLatestAccounts) accList(result) {
this.wiredAccountList = result;
if (result.data) {
this.accountList = result.data;
this.error = undefined;
} else if (result.error) {
this.error = result.error;
this.accountList = [];
}
}
handelSelection(event) {
if (event.detail.selectedRows.length > 0) {
this.selectedRecord = event.detail.selectedRows[0].Id;
}
}
deleteRecord() {
deleteRecord(this.selectedRecord)
.then(() => {
refreshApex(this.wiredAccountList);
})
.catch(error => {
})
}
}
<template>
<lightning-card title="Latest Five Accounts">
<lightning-button slot="actions" label="Delete Account" onclick={deleteRecord}></lightning-button>
<lightning-datatable
data={accountList} columns={cols} key-field="Id"
max-row-selection="1" onrowselection={handelSelection} >
</lightning-datatable>
</lightning-card>
</template>
<<<<<<AURA Interview Questions>>>>>>
How to fix above error:-
1. What is Lightning Component Framework and What is Aura Framework?
- Lightning Component framework is a UI framework for developing single page applications for mobile and desktop devices.
- It uses JavaScript on the client side and Apex on the server side.
- Lightning Component framework makes developing apps that work on both mobile and desktop devices far simpler than many other frameworks.
- Lightning component Framework is based on Aura Framework.
- Aura Framework is design by Salesforce which is open source framework for creating ui components.
2. What is Lightning App Builder?
Lightning app builder is used to create lightning pages for Salesforce Lightning experience and mobile apps.The Lightning App Builder is a point-and-click tool.Lightning Pages are built using Lightning components which are compact, configurable, and reusable elements that you can drag and drop into regions of the page in the Lightning App Builder.
We can create different types of pages using lightning app builder,
1)App Page
2)Home Page
3)Record Page
3. What is the use of $A.enqueueAction(action) and how to call server side action from client side?
$A.enqueueAction(action) adds the server-side controller action to the queue of actions to be executed.The actions are asynchronous and have callbacks.
4. What are Attributes in Salesforce Lightning component?
Attributes like a variable to store different types of values.
5. What are collection types in Salesforce Lightning component?
- Array
- List
- Map
- Set
6. What is the use of interface force:hasRecordId?
By using force:hasRecordId interface in lightning component we can assigned the id of the current record to lightning component. This interface adds an attribute named “recordId” to the component. The type of attribute is string and has 18-character Salesforce record ID.
To make our component available for record page and any other type of page, we need to implement the interface flexipage:availableForAllPageTypes
7. What is the use of interface Lightning:actionOverride in Salesforce Lightning component?
Lightning:actionOverride interface Enables a component to be used as an override for a standard action. We can override the View,New, Edit, and Tab standard actions on most standard and all custom components. The component needs to implement the interface Lightning:actionOverride after which the component appear in the Lightning Component Bundle menu of an object action Override Properties panel.
8. Do we need custom domain to create Lightning components?
No, we can create them without custom domain but to view them we need custom domain
9. Do we need custom namespaces to develop lightning components?
No, It’s Optional.
10. Can we access one lightning component inside another lightning component in salesforce?
Yes, we can
11. Can we access one JavaScript controller method on another controller method in salesforce lightning component?
No, we cannot access them
12. Can we access one JavaScript helper method on another helper method in lightning Component?
Yes, we can access using this
keyword.
testMethod1 : function (component, param){ //do stuff } testMethod2: function (component, params){ //do stuff, and this.testMethod1(component, params) }
13. Can we use external libraries in components?
14. How we can enable component for lightning Tabs?
By implementing interface implements=”force:appHostable”.
15. How we can enable component for lightning Record home page?
Record pages are different from app pages in a key way: they have the context of a record. To make your components display content that is based on the current record, use a combination of an interface and an attribute.
- If your component is available for both record pages and any other type of page, implement
flexipage:availableForAllPageTypes
. - If your component is designed only for record pages, implement the
flexipage:availableForRecordHome
interface instead offlexipage:availableForAllPageTypes
. - If your component needs the record ID, also implement the force:hasRecordId interface.
- If your component needs the object’s API name, also implement the force:hasSObjectName interface.
16. How we can enable component for lightning action?
By implementing interface implements=”force:lightningQuickAction”
17. What are Naming Convention Rule for Salesforce Lightning?
A component name must follow these naming rules:
- Must begin with a letter
- Must contain only alphanumeric or underscore characters
- Must be unique in the namespace
- Can’t include whitespace
- Can’t end with an underscore
- Can’t contain two consecutive underscores
18. How to use static resources in Lightning Components/Application?
Following steps are used to load the external Java Script library in lightning component.
- Load your external java script library in static resource. Goto >> SetUp>> Custom Code >> Static Resources
- Use <ltng:require> tag to load the Java Script library in lightnig component as follows
<!-- For one js library -->
<!-- Here filename1 is static resource name -->
<ltng:require scripts="{!$Resorce.filename1}"></ltng:require>
<!-- For more than one js file -->
<!-- Here filename1 and filename2 is static resource name -->
<ltng:require scripts="{!join(',',
$Resource.filename1,
$Resource.filename2)}"></ltng:require>
19. What are value providers in Salesforce Lightning?
Value providers are a way to access data. Value providers encapsulate related values together, similar to how an object encapsulates properties and methods.
The value providers for a component are v (view) and c (controller).
20. List of Global value providers ?
- $globalID
- $Browser
- $ContentAsset
- $Label
- $Locale
- $Resource
21. How we can access Custom Label in Lightning?
Syntax : $A.get(“$Label.namespace.labelName”)
22. Where can we use lightning component in LEX?
- Lightning Pages and Lightning App Builder
- Lightning Community builder
- Lightning Flow
- In Visualforce
23. What is Lightning Container?
Use lightning:container to use third-party frameworks like AngularJS or React within your Lightning pages. Upload an app developed with a third-party framework as a static resource, and host the content in an Aura component using lightning:container.
24. What is Lightning Locker?
Lightning Locker is a powerful security architecture for Lightning components. Lightning Locker enhances security by isolating Lightning components that belong to one namespace from components in a different namespace. Lightning Locker also promotes best practices that improve the supportability of your code by only allowing access to supported APIs and eliminating access to non-published framework internals.
25. What is Value provider and What is action provider in Salesforce Lightning component?
Value provider is a way to access data,with value provider “v” we can fetch value of attribute from component markup in javascript controller.Value provider is denoted with v(view) and action provider is denoted with “c “(controller).
Value provider:
This value provider enable us to access value of component attribute in component markup and javascript controller.
<aura:component>
<aura:attribute type="string" name="myfirstattribute" default="first text"/>
{!v.myfirstattribute}
</aura:component>
Action provider:
This enable us to handle actions,event,handlers for the component.
<aura:handler name="init" value="{!this}" action="{!c.doinit}"/>
({
doinit:function(component, event, helper) {
// some operations here
}
})
26. What is the use of interface flexipage:availableForRecordHome in Salesforce Lightning component?
Interface flexipage:availableForRecordHome make the component available for record pages only.
27. What is the use of interface force:appHostable in Salesforce Lightning component?
Interface force:appHostable in component allow it to be used as a custom tab in Lightning Experience or the Salesforce mobile app.
28. What is the difference between force:lightningQuickAction and force:lightningQuickActionWithoutHeader in Salesforce Lightning component?
Interface force:lightningQuickAction allow the component to be used as a custom action in Lightning Experience or the Salesforce mobile app. Implementing the force:lightningQuickAction in a component, component interface display in a panel with standard action controls, such as a Cancel button.
Interface force:lightningQuickActionWithoutHeader allow the component to be used as a custom action in Lightning Experience or the Salesforce mobile app. Implementing the force:lightningQuickAction in a component, component interface display in a panel without standard action controls like cancel button.
29. What is the use of aura:handler init event in Salesforce Lightning component?
This is also called as the constructor of lightning component. The init event is called once the component construction is over. As an example if I am creating a simple registration page and I want to display some default text value after page load in my input boxes I can do this by calling the javascript controller method and setting some default value in the attributes.
<aura:handler name="init" value="{!this}" action="{!c.doinit}"/>
30. What is the use of aura:handler change event in the Salesforce Lightning component?
The change event is called when the value in one of the attribute changes.As an Example If i am having attribute ” Anyname”, on change of attribute “Anyname” if I want to call javascript method i can do this as:
<aura:attribute name="Anyname" type="String" />
<aura:handler name="change" value="{!v.Anyname}" action="{!c.doinit}"/>
31. What is component event in Salesforce Lightning component?
Component Events are fired by the child components and handled by the parent component.We require parent child relationship between two components if we want to use component event between them for communication.
32. What is application event in Salesforce Lightning component?
Application event are the event handle by any component no matter what relationship between them but they should be inside single application.
33. What is the main advantage of using Component event over application events?
We should always use Component Events whenever possible this is because Component events can only be handled by parent components so the components in picture are only those components which need to know about them.
34. Explain Bound and Unbound expressions in Salesforce Lightning component?
We have two types of expression Bound expression and Unbound expression which we use to perform data binding in lightning components.
<c:ChildComp childAttributeName="{!v.parentAttributeName}" /> <!--Bound Expression-->
childAttributeName=”{!v.parentAttributeName}” is a bound expression. Any change to the value of the childAttributeName attribute in child component also changes.
<c:ChildComp childAttributeName="{#v.parentAttributeName}" /> <!--Unbound Expression-->
childAttributeName=”{#v.parentAttributeName}” is a Unbound expression. Any change to the value of the childAttributeName attribute in child component has no effect.
35. What is Aura:method in Salesforce Lightning component?
we can directly call a child component controller method from the parent component controller method using Aura:method. This method is used to pass value from parent component controller to the child component controller.
36. What is Lightning:overlayLibrary in Salesforce Lightning component?
To create a modal box we use Lightning:overlayLibrary.To use Lightning:overlayLibrary in component we need to include tag <lightning:overlayLibrary aura:id=”overlayLib”/> in component, here aura:id is unique local id. Modal has header,body and footer which are customizable.
37. What is lightning:navigation in Salesforce Lightning component?
Lightning:navigation is used to navigate to a given pageReference.
Following are the supported type where we can navigate,
Lightning Component
Knowledge Article
Named Page
Navigation Item
Object Page
Record Page
Record Relationship Page
Web Page
Please visit this link for more details:- https://developer.salesforce.com/docs/component-library/bundle/lightning: avigation/documentation
38. How access parent component method by Child component without Event?
You can pass a reference to the parent into the child, so in the parent component:
<aura:method name="parentMethod" .../>
<c:child parent="{!this }" ... />
and in the child component you can then invoke the parent method:
<aura:attribute name="parent" type="Aura.Component" required="true"/>
someMethod: function(component, event, helper) {
var p = component.get("v.parent");
p.parentMethod();
}