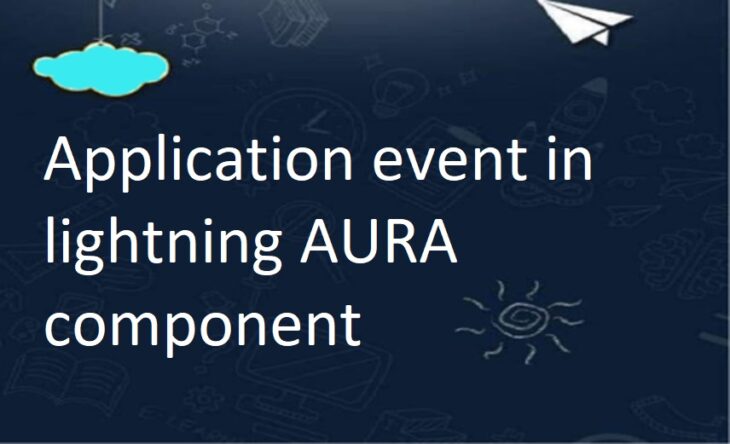
Application event in Lightning AURA component
Lightning framework is based on event-driven architecture which allows to communicate between different events. Lightning events are fired from JavaScript controller actions that are triggered by a user interacting with the user interface.
Note:-The handler for an application event won’t work if you set the name attribute in <aura:handler>. Use the name attribute only when you’re handling component events.
The communication between components are handled by events. There are two types of custom events in the Lightning Framework:
- Component Events
- Application Events
Application Events are handled by any component have handler defined for event.These events are essentially a traditional publish-subscribe model. link
Application Event:
SampleApplicationEvent.evt
<!--SampleApplicationEvent.evt-->
<aura:event type="Application" description="Sample Application Event">
<aura:attribute name="message" type="String" />
</aura:event>
Component1.cmp:
<!--Component1.cmp-->
<aura:component>
<aura:registerEvent name="SampleApplicationEvent" type="c:SampleApplicationEvent"/>
<lightning:button label="Click to fire the event" variant="brand" onclick="{!c.component1Event}"/>
</aura:component>
Component1 JS Controller: Use $A.get(“e.myNamespace:myAppEvent”) in JavaScript to get an instance of the myAppEvent
event in the myNamespace
namespace.
To set the attribute values of event, call event.setParam()
or event.setParams()
.
({
component1Event : function(cmp, event,helper) {
//Get the event using event name.
var appEvent = $A.get("e.c:SampleApplicationEvent");
//Set event attribute value
appEvent.setParams({"message" : "Welcome "});
appEvent.fire();
}
})
Component2: The application event handled by the Component2 that fired using aura:handler
in the markup.
The action attribute of aura:handler
sets the client-side controller action to handle the event.
<!--Component2.cmp-->
<aura:component>
<aura:attribute name="eventMessage" type="String"/>
<aura:handler event="c:SampleApplicationEvent" action="{!c.component2Event}"/>
<div class="slds-m-around_xx-large">
<p>{!v.eventMessage}</p>
</div>
</aura:component>
Component2 JS Controller:
({
component2Event : function(cmp, event) {
//Get the event message attribute
var message = event.getParam("message");
//Set the handler attributes based on event data
cmp.set("v.eventMessage", message + 'Biswajeet');
}
})
Lightning Application:
<aura:application extends="force:slds">
<c:Component1/>
<c:Component2/>
</aura:application>