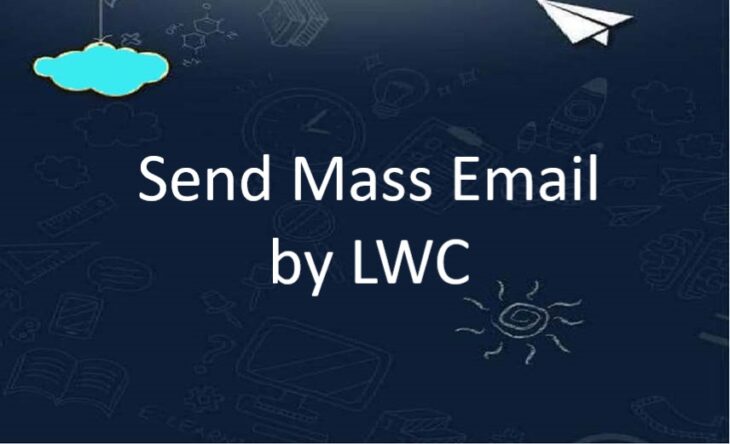
Send Mass Email By LWC
Send Mass Email By Lightning Web Component:-
This component will use to send email to selected Contacts, by Lightning web component , just for testing i have drag this component on Account record page
sendMassEmail.html:-
<template>
<lightning-card variant="Narrow" title="Contact List" icon-name="standard:contact">
<lightning-button variant="Neutral" label="Send Email" onclick={handleClick}></lightning-button>
<lightning-datatable
data={data}
columns={column}
key-field="id"
onrowselection={getSelectedName}
>
</lightning-datatable>
</lightning-card>
</template>
sendMassEmail.js:-
import { LightningElement,wire } from 'lwc';
import getContactList from '@salesforce/apex/SendMassEmail.getContactRecords';
import sendEmailToContact from '@salesforce/apex/SendMassEmail.sendEmails';
const columns = [
{ label: 'Id', fieldName: 'Id', type: 'text' },
{ label: 'Name', fieldName: 'Name', type: 'text' },
{ label: 'Email', fieldName: 'Email'}
];
import {ShowToastEvent} from 'lightning/platformShowToastEvent';
export default class SendEmialFromLWC extends LightningElement {
data;
error;
listOfContacts = [];
column = columns;
@wire(getContactList)
wiredClass({data, error}) {
if(data) {
this.data = data;
}
else if(error) {
this.error = error;
this.data = undefined;
}
}
getSelectedName(event) {
const selectedRows = event.detail.selectedRows;
for (let i = 0; i < selectedRows.length; i++) {
this.listOfContacts.push(selectedRows[i].Id);
}
}
handleClick(){
sendEmailToContact({
ConList: this.listOfContacts
})
.then(result =>{
var toast = new ShowToastEvent({
'title': 'Successfull',
'message': 'Email Sent Successfully',
'variant': 'success'
});
this.dispatchEvent(toast);
}).catch(error =>{
console.log(error);
})
}
}
sendMassEmail.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>52.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
<target>lightning__AppPage</target>
<target>lightning__HomePage</target>
</targets>
<targetConfigs>
<targetConfig targets="lightning__RecordPage">
<objects>
<object>Account</object>
</objects>
</targetConfig>
</targetConfigs>
</LightningComponentBundle>
SendMassEmail.cls
public with sharing class SendMassEmail {
@AuraEnabled(cacheable = true)
public static List<Contact> getContactRecords(){
return [select ID,Name,Email from Contact limit 10];
}
@AuraEnabled(cacheable = true)
public static void sendEmails(List<String> ConList){
// Step 0: Create a master list to hold the emails we'll send
List<Messaging.SingleEmailMessage> mails = new List<Messaging.SingleEmailMessage>();
For(Contact con : [Select Id, FirstName, Email from Contact where Id IN: ConList]){
Messaging.SingleEmailMessage mail = new Messaging.SingleEmailMessage();
// Step 1: Set list of people who should get the email
List<String> sendTo = new List<String>();
sendTo.add(con.Email);
mail.setToAddresses(sendTo);
mail.setSenderDisplayName('Official Bank of Notification');
// Step 3. Set email contents - you can use variables!
mail.setSubject('URGENT BUSINESS PROPOSAL');
String body = 'Dear ' + con.FirstName + ', ';
body += 'Please update your permanent address.';
body += 'our sales person getting diffecultis to found your address';
mail.setHtmlBody(body);
mails.add(mail);
}
// Step 4: Send all emails in the master list
if(mails.size() > 0) {
try{
Messaging.sendEmail(mails);
}catch(exception e){
System.debug('getMessage-->>>>>> '+e.getMessage());
}
}
}
}