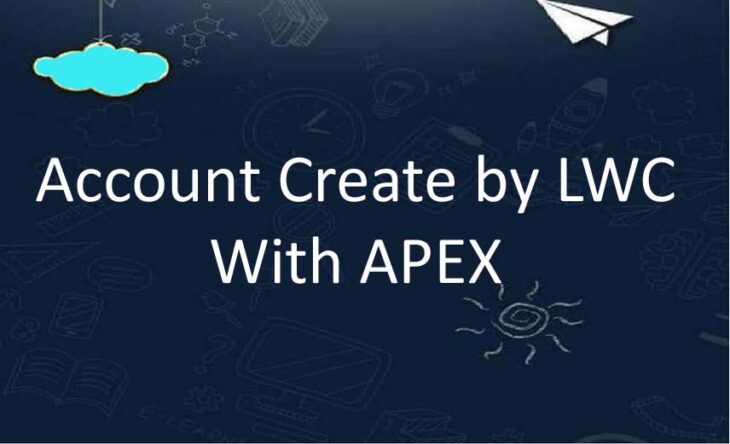
Account Record Insert using Apex class in LWC
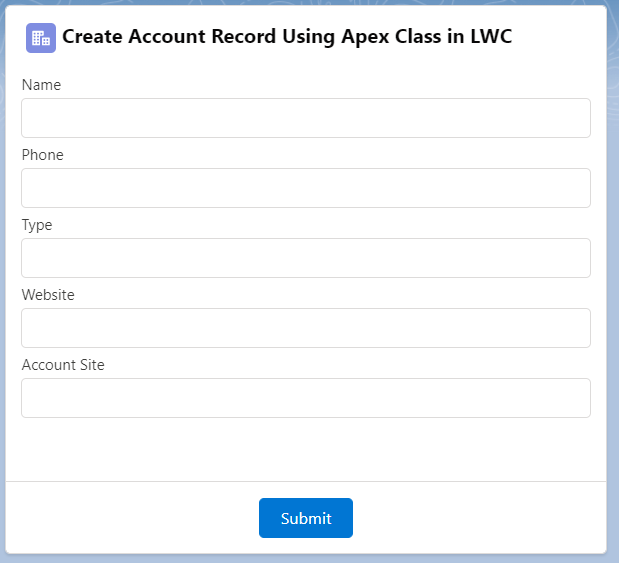
insertAccountByApex.html
<template>
<lightning-card>
<div slot="title">
<h3>
<lightning-icon icon-name="standard:account" size="small"></lightning-icon> Create Account Record Using Apex Class in LWC
</h3>
</div>
<p class="slds-p-horizontal_small">
<lightning-input type="text" name="name" label="Name" value={getAccountDetail.Name} onchange={changeHandler}></lightning-input>
</p>
<p class="slds-p-horizontal_small">
<lightning-input type="text" name="phone" label="Phone" value={getAccountDetail.Phone} onchange={changeHandler}></lightning-input>
</p>
<p class="slds-p-horizontal_small">
<lightning-input type="text" name="type" label="Type" value={getAccountDetail.Type} onchange={changeHandler}></lightning-input>
</p>
<p class="slds-p-horizontal_small">
<lightning-input type="text" name="website" label="Website" value={getAccountDetail.Website} onchange={changeHandler}></lightning-input>
</p>
<p class="slds-p-horizontal_small">
<lightning-input type="text" name ="site" label="Account Site" value={getAccountDetail.Site} onchange={changeHandler}></lightning-input>
</p>
<div slot="footer">
<lightning-button label="Submit" onclick={saveAccountAction} variant="brand"></lightning-button>
</div>
<br/> <br/>
</lightning-card>
</template>
insertAccountByApex.js
import { LightningElement,track } from 'lwc';
import createAccountRecord from '@salesforce/apex/InsertAccountByApexController.insertAccount';
import accountName from '@salesforce/schema/Account.Name';
import accountPhone from '@salesforce/schema/Account.Phone';
import accountType from '@salesforce/schema/Account.Type';
import accountWebsite from '@salesforce/schema/Account.Website';
import accountSite from '@salesforce/schema/Account.Site';
import {ShowToastEvent} from 'lightning/platformShowToastEvent';
export default class InsertAccountByApex extends LightningElement {
@track accountid;
@track error;
@track getAccountDetail={
Name:accountName,
Phone:accountPhone,
Type:accountType,
Website:accountWebsite,
Site:accountSite
};
changeHandler(event){
if(event.target.name == 'name'){
this.getAccountDetail.Name = event.target.value;
}
if(event.target.name == 'phone'){
this.getAccountDetail.Phone = event.target.value;
}
if(event.target.name == 'site'){
this.getAccountDetail.Site = event.target.value;
}
if(event.target.name == 'type'){
this.getAccountDetail.Type = event.target.value;
}
if(event.target.name == 'website'){
this.getAccountDetail.Website = event.target.value;
}
}
saveAccountAction(){
createAccountRecord({accountObj:this.getAccountDetail})
.then(result=>{
this.getAccountDetail={};
this.accountid=result.Id;
window.console.log('New Account Created Id : ' + this.accountid);
const toastEvent = new ShowToastEvent({
title:'Success!',
message:'New Account successfully',
variant:'success'
});
this.dispatchEvent(toastEvent);
})
.catch(error=>{
this.error=error.message;
window.console.log(this.error);
});
}
}
insertAccountByApex.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>45.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__AppPage</target>
<target>lightning__RecordPage</target>
<target>lightning__HomePage</target>
</targets>
</LightningComponentBundle>