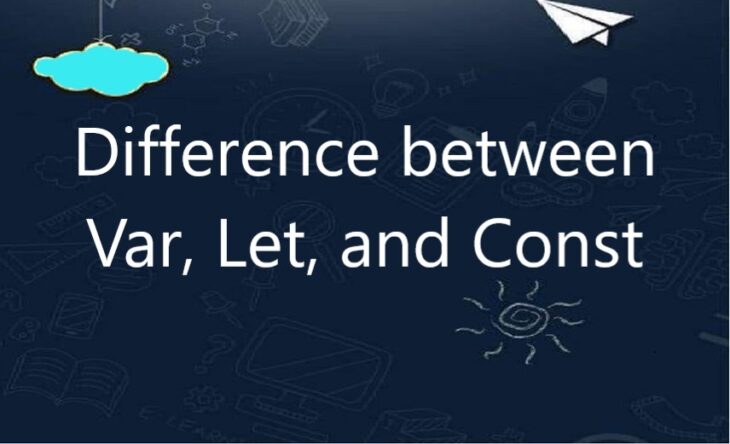
Difference between Var, Let, and Const in LWC
One of the features that came with ES6 is the addition of let
and const
, which can be used for variable declaration.
<<<<<<<<<<<<<<<Scope of var>>>>>>>>>>>>>>>
Scope essentially means where these variables are available for use. var
declarations are globally scoped or function/locally scoped.
The scope is global when a var
variable is declared outside a function. This means that any variable that is declared with var
outside a function block is available for use in the whole window.
var test1 = "hey hi";
function newFunction() {
var test2 = "hello";
}
Here, test1
is globally scoped because it exists outside a function while test2
is function scoped. So we cannot access the variable test2
outside of a function. So if we do this:
var test 1 = "hey hi";
function newFunction() {
var test2 = "hello";
}
console.log(test2); // error: test2 is not defined
<<<<<<<<<<<<<<Problem with var>>>>>>>>>>>>>
var test1 = "hey hi";
var times = 4;
if (times > 3) {
var test1 = "say Hello instead";
}
console.log(test1) // "say Hello instead" It's showing local variable instead of global variable
If you have used test1
in other parts of your code, you might be surprised at the output you might get. This will likely cause a lot of bugs in your code. This is why let
and const
are necessary.
var variables can be re-declared and updated
This means that we can do this within the same scope and won’t get an error.
var test1= "hey hi";
var test1= "say Hello instead";
<<<<<<<<<<<<<<<Scope of Let>>>>>>>>>>>>>>>
let
is now preferred for variable declaration. It’s no surprise as it comes as an improvement to var
declarations. It also solves the problem with var
that we just covered. Let’s consider why this is so.
So a variable declared in a block {} with let
is only available for use within that block. Let me explain this with an example:
let greeting = "say Hi";
let times = 4;
if (times > 3) {
let test1 = "say Hello instead";
console.log(test1);// "say Hello instead"
}
console.log(test1) // test1 is not defined
We see that using test1
outside its block (the curly braces where it was defined) returns an error. This is because let
variables are block scoped .
<<<let can be updated but not re-declared>>>
Just like var
, a variable declared with let
can be updated within its scope. Unlike var
, a let
variable cannot be re-declared within its scope. So while this will work:
let test1 = "say Hi";
test1 = "say Hello instead"
this will return an error:
let test1= "say Hi";
let test1= "say Hello instead"; // error: Identifier 'test1' has already been declared
However, if the same variable is defined in different scopes, there will be no error:
let test1 = "say Hi";
if (true) {
let test1 = "say Hello instead";
console.log(test1); // "say Hello instead"
}
console.log(test1); // "say Hi"
This fact makes let
a better choice than var
. When using let
, you don’t have to bother if you have used a name for a variable before as a variable exists only within its scope.
<<<<<<<<<Const>>>>>>>>>>>
Like let
declarations, const
declarations can only be accessed within the block they were declared.
const cannot be updated or re-declared
This means that the value of a variable declared with const
remains the same within its scope. It cannot be updated or re-declared. So if we declare a variable with const
, we can neither do this:
const test1 = "say Hi";
test1 = "say Hello instead";// error: Assignment to constant variable.
const test1 = "say Hi";
const test1 = "say Hello instead";// error: Identifier 'greeting' has already been declared