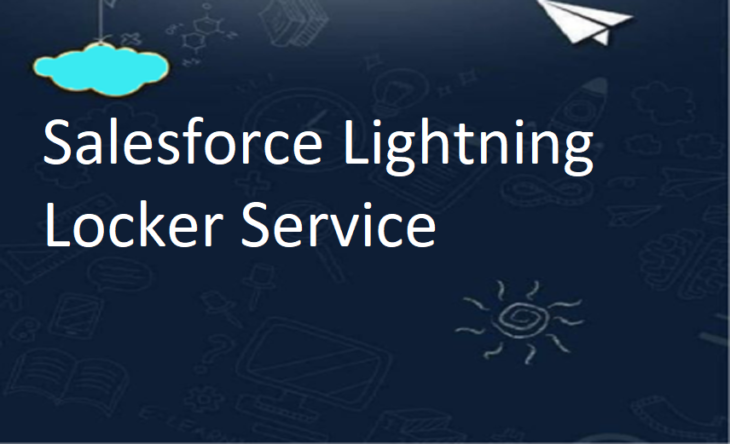
Salesforce Lightning Locker Service
What is Lightning Locker Service? Salesforce link
- Enforces powerful security architecture in single page applications built using lighting components.
- Isolates the components belonging to one namespace from the components belonging to other namespaces.
- Promotes lighting best practices by allowing access to only supported API’s and prevent the execution of malicious code in a trusted webpage context.
- Lighting locker uses a browser Content Security Policy (CSP) which prevents the webpage against Cross-Site Scripting (XSS), Click-Jacking and other code injection.
Why do we need ‘Security’ in Lightning?
- For protection of the web pages built using lighting components against web security vulnerabilities.
- To make the component have access to only the DOM that was created by our component code
- Locker Services adds namespaces to our components which prevent the component code from accessing data directly from the components of other namespaces.
Salesforce Content Security Policy (CSP):-
Salesforce’s Content Security Policy doesn’t allow the use of inline JavaScript code and restricts the JavaScript eval() to the global namespace.
Strict Mode Enforcement in JS:-
Locker Service automatically enforces the ECMAScript (ES5) strict mode in our JS code. If we write a JS code that is not valid in strict mode, we will see unexpected errors. If we are using external JS libraries, we need to ensure that they are also following the strict mode.
best practices to be followed while writing the code in Lightning Component Controller.js and Helper.js files are listed below:
1. Using a variable/object without declaring is not allowed:
({
myAction : function(component, event, helper) {
amount = 100 // will through error,because we don't declare
}
})
({
myAction : function(component, event, helper) {
var amount = 100 // Correct
}
})
2. Writing to a read-only/get-only properties is not allowed
3. The following reserved keywords are not allowed in strict mode:
implements
· interface
· let
· package
· private
· protected
· public
· static
Let’s look at a sample component that demonstrates DOM containment.
<!--c:domLocker-->
<aura:component>
<div id="myDiv" aura:id="div1">
<p>See how Lightning Locker restricts DOM access</p>
</div>
<lightning:button name="myButton" label="Peek in DOM"
aura:id="button1" onclick="{!c.peekInDom}"/>
</aura:component>
The c:domLocker component creates a <div> element and a lightning:button component.
Here’s the client-side controller that peeks around in the DOM.
({ /* domLockerController.js */
peekInDom : function(cmp, event, helper) {
console.log("cmp.getElements(): ", cmp.getElements());
// access the DOM in c:domLocker
console.log("div1: ", cmp.find("div1").getElement());
console.log("button1: ", cmp.find("button1"));
console.log("button name: ", event.getSource().get("v.name"));
// returns an error
//console.log("button1 element: ", cmp.find("button1").getElement());
}
})
Valid DOM Access:-
The following methods are valid DOM access because the elements are created by c:domLocker.
cmp.getElements()
Returns the elements in the DOM rendered by the component.
cmp.find()
Returns the div and button components, identified by their aura:id attributes.
cmp.find("div1").getElement()
Returns the DOM element for the div as c:domLocker created the div.
event.getSource().get("v.name")
Returns the name of the button that dispatched the event; in this case, myButton.
Invalid DOM Access:-
You can’t use cmp.find(“button1”).getElement() to access the DOM element created by lightning:button. Lightning Locker doesn’t allow c:domLocker to access the DOM for lightning:button because the button is in the lightning namespace and c:domLocker is in the c namespace.
Enable the Locker Service and select the Locker API Version:
In order to enable the Locker Service for a component, choose the Bundle Version to 40.0 or higher and the Locker Service will automatically be enforced. Similarly, if we want to disable this for a lightning component, we can set the API Version to 39.0 or lower but it is not recommended to do so.
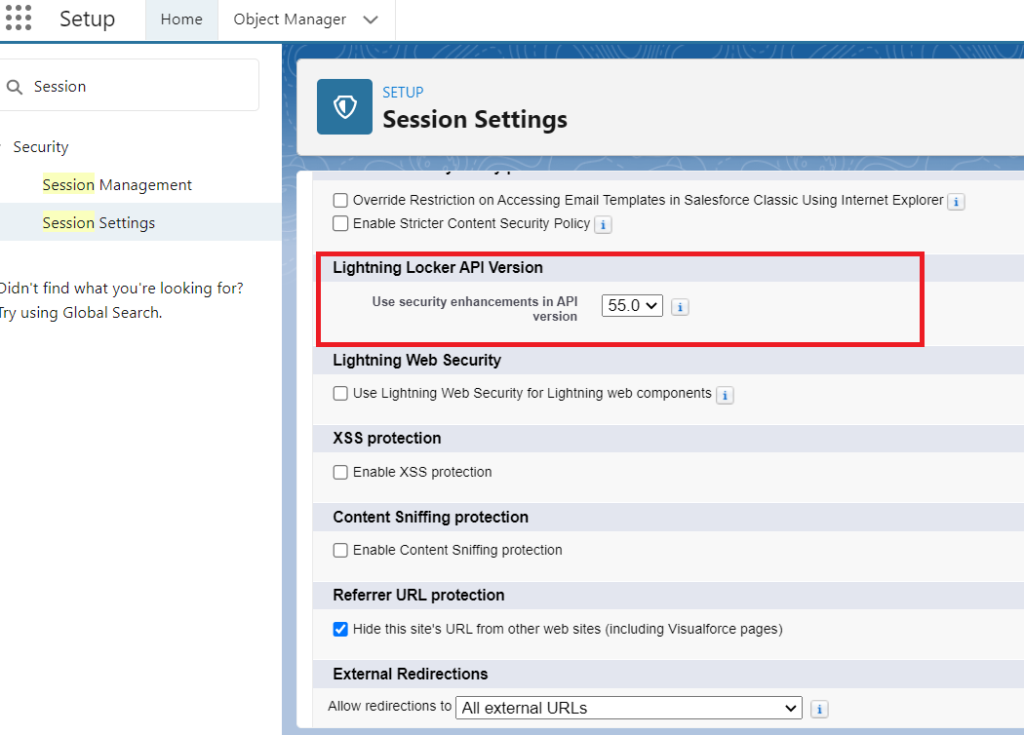