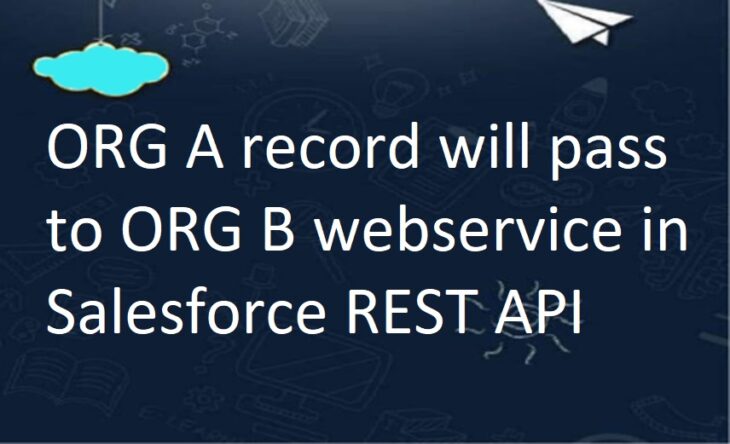
ORG A record will pass to ORG B webservice in Salesforce REST API
Just taking about live scenario, For now we have two ORG, ORG A and another ORG B. ORG A record will pass to ORG B webservice, The webservice will than create the same record in ORG B.
Rest api is a simple and power webservice based on restful principles and it uses rest resource and HTTP methods to expose functionalities. It supports both XML and JSON. Rest api has lightweight requests and responsive framework and it is good for mobile and web apps.
In this scenario ORG B(TARGET) and ORG A(SOURCE)
What steps we need to do on ORG B :-
- Create a connected app.
- Rest api webservice to create data based on the requirement of ORG A.
REST API WEBSERVICE FROM ORG B:
@RestResource(urlMapping='/createAccountRecord/*')
global with sharing class createAccountRecord {
@HttpPost
global Static string createAccount(){
RestRequest req = RestContext.request;
RestResponse res = Restcontext.response;
string jsonString=req.requestBody.tostring();
responseWrapper wResp=(responseWrapper) JSON.deserialize(jsonString,responseWrapper.class);
Account obj=new Account();
obj.Name=wResp.name;
Insert obj;
return 'Success';
}
global class responseWrapper{
global string name;
}
}
What steps we need to do on ORG A :-
- Create a apex trigger.
- Create an apex controller.
- Create a remote site setting for the URL of System B.
When we try to create a new account in ORG A then trigger will execute callout method with Authtication
Trigger in ORG A
trigger Accounttrigger on Account (after insert) {
Set<id> accIdSet=new Set<id>();
for(Account acc:trigger.new){
if(acc.name!=Null){
accIdSet.add(acc.id);
}
}
if(accIdSet.size() > 0){
callWebserviceClass.getConList(accIdSet);
}
}
APEX CONTROLLER FROM ORG A :
public class callWebserviceClass {
private string cKey = 'XXXXXXXXXXXXXXXXXXXXXXXXXXX';
private string cSecret = 'XXXXXXXXXXXXXXXXXXXXXXXXXXX';
private string uName = 'XXXXXXXXXXXXXXXXXXXXXXXXXXX';
private string passwd = 'Password+SecurityToken';
public class responseWrapper {
public string id;
public string access_token;
public string instance_url;
}
public string getRequestToken() {
string reqBody = 'grant_type=password&client_id=' + cKey + '&client_secret=' + cSecret + '&username=' + uName + '&password=' + passwd;
Http h = new Http();
HttpRequest req = new HttpRequest();
req.setBody(reqBody);
req.setMethod('POST');
req.setEndpoint('https://login.salesforce.com/services/oauth2/token');
HttpResponse hresp = h.send(req);
responseWrapper wResp = (responseWrapper) JSON.deserialize(hresp.getBody(), responseWrapper.class);
system.debug('Instance url' + wResp.instance_url);
system.debug('session id' + wResp.access_token);
return wResp.access_token;
}
@future(callout = true)
public static void getConList(Set < id > accIdSet) {
String accToken;
string responseBody;
string endPoint = 'https://ap5.salesforce.com/services/apexrest/createAccountRecord';
callWebserviceClass obj = new callWebserviceClass();
accToken = obj.getRequestToken();
system.debug('access token' + accToken);
if (accToken != '') {
for (Account acc: [SELECT id,name from account where id in: accIdSet]) {
system.debug('JSON' + JSON.serialize(acc));
Http h1 = new Http();
HttpRequest req1 = new HttpRequest();
req1.setHeader('Authorization', 'Bearer ' + accToken);
req1.setHeader('Content-Type', 'application/json');
//req1.setHeader('accept','application/json');
req1.setMethod('POST');
req1.setBody(JSON.serialize(acc));
req1.setEndpoint(endPoint);
HttpResponse hresp1 = h1.send(req1);
system.debug('hresp1' + hresp1);
}
}
}
}