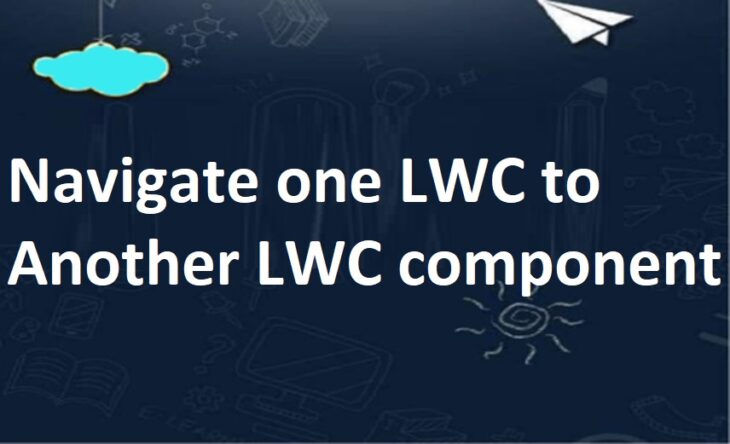
How to navigate one LWC Component to Another LWC Component
Currently LWC doesn’t support component navigation like LWC to LWC. so for this solution we need to create Aura Component as a middle-ware that’s navigate to another LWC.
This Aura Component having lightning:isUrlAddressable is added as interface. To enable direct navigation to a Lightning component via URL, add the lightning:isUrlAddressable
interface to the component.
So we will create two lightning web component and one Aura component to test this feature:-
sourceLWC.html
<template>
<lightning-card title="Source LWC">
<lightning-button name="navigateToTargetLWC" onclick= {handleTargetLwc} label="navigateToTargetLWC">navigateToTargetLWC</lightning-button>
</lightning-card>
</template>
sourceLWC.js
import { LightningElement } from 'lwc';
import { NavigationMixin } from 'lightning/navigation';
export default class SourceLWC extends NavigationMixin (LightningElement) {
handleTargetLwc(event){
this[NavigationMixin.Navigate]({
type: "standard__component",
attributes: {
componentName: "c__middleWareAura"
},
state: {
c__productName: 'LG 5* Split AC',
c__productPrice: '50000',
c__productQuentity: '1'
}
})
}
}
sourceLWC.js-meta.xml
<?xml version="1.0" encoding="UTF-8"?>
<LightningComponentBundle xmlns="http://soap.sforce.com/2006/04/metadata">
<apiVersion>55.0</apiVersion>
<isExposed>true</isExposed>
<targets>
<target>lightning__RecordPage</target>
</targets>
</LightningComponentBundle>
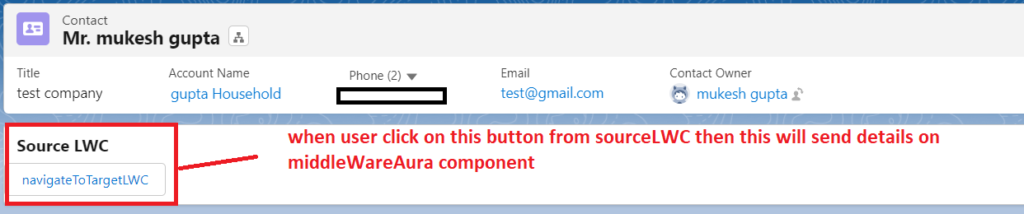
Below Aura Component will embed targetLWC component for navigation
middleWareAura.cmp
<aura:component implements="flexipage:availableForAllPageTypes,lightning:isUrlAddressable" access="global">
<aura:attribute type="String" name="pName"/>
<aura:attribute type="String" name="pPrice"/>
<aura:attribute type="String" name="pQuentity"/>
<div><p style="font-size: 30px;color: white;">Your Product Details:-</p></div>
<aura:handler name="init" value="{!this}" action="{!c.init}"/>
<div class="slds-card">
<c:targetLWC pName = "{!v.pName}" pPrice = "{!v.pPrice}" pQuentity = "{!v.pQuentity}"/>
</div>
</aura:component>
middleWareAuraController.js
({
init : function(component, event, helper) {
var myPageRef = component.get("v.pageReference");
var propertyName = myPageRef.state.c__productName;
var propertyPrice = myPageRef.state.c__productPrice;
var propertyQuentity = myPageRef.state.c__productQuentity;
component.set("v.pName", propertyName);
component.set("v.pPrice", propertyPrice);
component.set("v.pQuentity", propertyQuentity);
}
})
Now targetLWC component will display information that’s sending from sourceLWC component
targetLWC.html
<template>
productName:- {pName} <br>
productPrice:- {pPrice}<br>
productQuentity:- {pQuentity}
</template>
targetLWC.js
import { api, LightningElement } from 'lwc';
export default class TargetLWC extends LightningElement {
@api pName;
@api pPrice;
@api pQuentity;
connectedCallback(){
}
}
According to below screen, middleWareAura component sent data to targetLWC that’s showing on screen
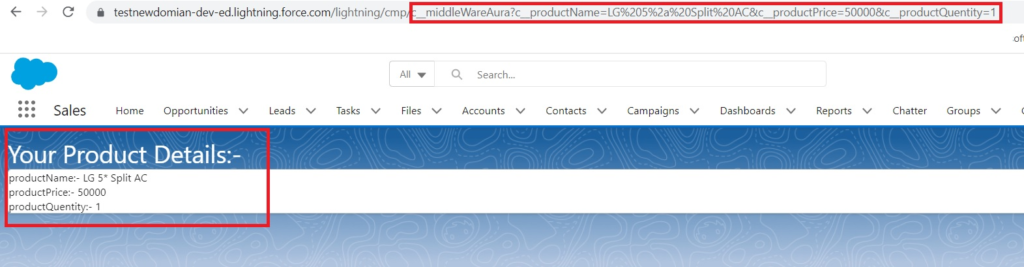