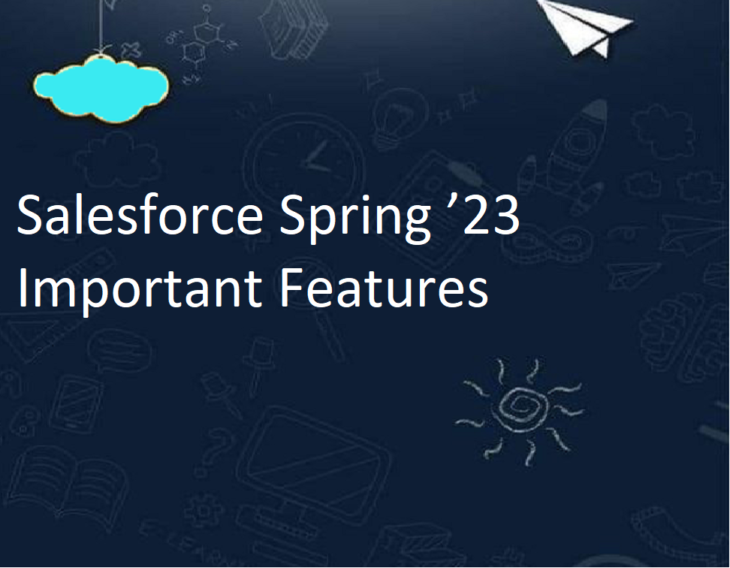
Salesforce Spring ’23 Important Features
Lightning Web Component(LWC)
1. Query DOM Elements with Refs:-
Now we can use refs to access elements in shadow DOM and light DOM, Refs locate DOM elements without a selector and only query elements contained in a specified template.
Previously, we was following only use querySelector()
to locate specific DOM elements.
Example:-
<template>
<div lwc:ref="myDiv"></div>
</template>
export default class extends LightningElement {
renderedCallback() {
console.log(this.refs.myDiv);
}
}
2. Use the Improved Conditional Directives
The lwc:if
, lwc:elseif
, and lwc:else
conditional directives supersede the legacy if:true
and if:else
directives.
Example:-
<template>
<template lwc:if={expression1}>
Statement 1
</template>
<template lwc:elseif={expression2}>
Statement 2
</template>
<template lwc:else>
Statement 3
</template>
</template>
Previously, chaining if:true
and if:false
blocks introduced performance cost and resulted in the property getters getting called multiple times.
<template>
<!---Replace if:true with lwc:if-->
<template if:true={isTemplateOne}>
This is template one.
</template>
<!-- Replace if:false with lwc:else -->
<template if:false={isTemplateOne}>
This is template two.
</template>
</template>
3. View Debug Information for Your Wired Properties
Access debug information for wired properties and methods on a component via custom formatters in Chrome DevTools.
Previously, to debug data received with a wired property, you had to use a wired function to return your data and inspect the deconstructed data
and error
properties.
import { LightningElement, wire } from 'lwc';
import getContactList from '@salesforce/apex/ContactController.getContactList';
export default class ApexWireMethodToProperty extends LightningElement {
@wire(getContactList) contacts;
}
please follow below url for more detail:-
4. New and Changed Modules for Lightning Web Components
lightning/uiRecordApi
:-
This module includes a new function:- notifyRecordUpdateAvailable(recordIds)
—Call this function to notify Lightning Data Service that a record has changed outside its mechanisms, such as via imperative Apex or by calling User Interface API via a third-party framework.
This module includes a deprecated function:- getRecordNotifyChange(recordIds)
(Deprecated)—Support for this wire adapter ends on May 1, 2024. We recommend using notifyRecordUpdateAvailable(recordIds)
instead.
APEX
1. Secure Apex Code with User Mode Database Operations
The new Database
and Search
methods support an accessLevel
parameter that lets you run database and search operations in user mode instead of in the default system mode.
You can indicate the mode of the operation by using WITH USER_MODE
or WITH SYSTEM_MODE
in your SOQL query. This example specifies user mode.
List<Account> acc = [SELECT Id FROM Account WITH USER_MODE];
Database operations can specify user or system mode. This example inserts a new account in user mode.
Account acc = new Account(Name='test');
insert as user acc;
2. Use the System.enqueueJob Method to Specify a Delay in Scheduling Queueable Jobs
Here are some cases where it can be beneficial to adjust the timing before the queueable job runs.
- If the external system is rate-limited and can be overloaded by chained queueable jobs that are making rapid callouts.
- When polling for results, and executing too fast can cause wasted usage of the daily async Apex limits.
This example adds a job for delayed asynchronous execution by passing in an instance of your class implementation of the Queueable
interface for execution. There’s a minimum delay of 5 minutes before the job is executed.
Integer delayInMinutes = 5;
ID jobID = System.enqueueJob(new MyQueueableClass(), delayInMinutes);
3. Dynamically Pass Bind Variables to a SOQL Query
With the new Database.queryWithBinds
, Database.getQueryLocatorWithBinds
, and Database.countQueryWithBinds
methods, the bind variables in the query are resolved from a Map parameter directly with a key rather than from Apex code variables.
In this example, the SOQL query uses a bind variable for an Account name. Its value (Acme Corporation
) is passed in using the acctBinds Map.
Map<String, Object> acctBinds = new Map<String, Object>{'acctName' => 'Acme Corporation'};
List<Account> accts =
Database.queryWithBinds('SELECT Id FROM Account WHERE Name = :acctName',
acctBinds,
AccessLevel.USER_MODE);