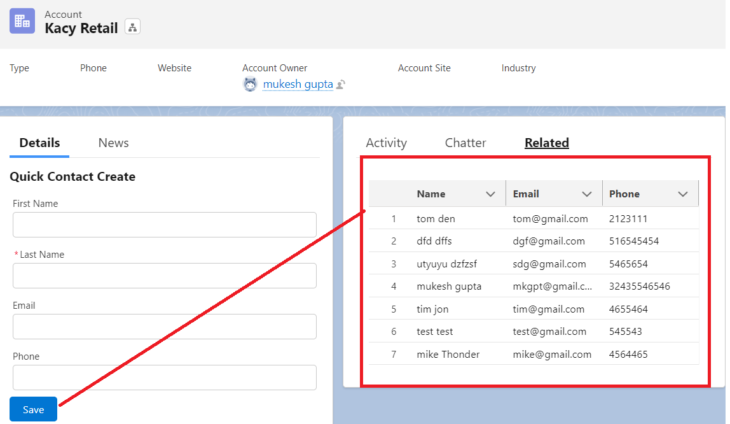
Create Related List in LWC by getRelatedListRecords
Salesforce provide a features for related list items. means we don’t need to write apex method to get child records of parent. just need to import getRelatedListRecords.
This blog will help to create a related list of Contact records, that’s will associate with particular Account record.
below component will create a contact and created contacts will be display in the contact’s Related list
contactsFromAccount.html
<template>
<lightning-card title="Quick Contact Create">
<lightning-record-edit-form object-api-name="Contact"
onsuccess={handleSuccess} onsubmit ={handleSubmit}>
<lightning-messages>
</lightning-messages>
<lightning-input-field field-name="FirstName">
</lightning-input-field>
<lightning-input-field field-name="LastName">
</lightning-input-field>
<lightning-input-field field-name="Email">
</lightning-input-field>
<lightning-input-field field-name="Phone">
</lightning-input-field>
<lightning-button
variant="brand"
type="submit"
name="save"
label="Save"
></lightning-button>
</lightning-record-edit-form>
</lightning-card>
</template>
contactsFromAccount.js
import { ShowToastEvent } from 'lightning/platformShowToastEvent';
import { LightningElement,api, wire } from 'lwc';
import FIRST_NAME_FIELD from '@salesforce/schema/Contact.FirstName';
import LAST_NAME_FIELD from '@salesforce/schema/Contact.LastName';
import ACCOUNT_ID_FIELD from '@salesforce/schema/Contact.AccountId';
import CONTACT_OBJECT from '@salesforce/schema/Contact';
export default class ContactsFromAccount extends LightningElement {
@api recordId;
objectApiName = CONTACT_OBJECT;
firstNameField = FIRST_NAME_FIELD;
lastNameField = LAST_NAME_FIELD;
AccountId = ACCOUNT_ID_FIELD;
handleSubmit(event) {
event.preventDefault();
const fields = event.detail.fields;
fields.AccountId = this.recordId;
this.template.querySelector('lightning-record-edit-form').submit(fields);
}
handleSuccess(event) {
const evt = new ShowToastEvent({
title: 'Contact Created',
message: 'Record ID: ' + event.detail.id,
variant: 'success',
});
this.dispatchEvent(evt);
}
}
Below is related list component:-
getRelatedContacts.html
<template>
<lightning-card>
<div class="slds-m-around_medium">
<lightning-datatable
key-field="Id"
data={records}
columns={columns}
hide-checkbox-column="true"
show-row-number-column="true">
</lightning-datatable>
</div>
</lightning-card>
</template>
getRelatedContacts.js
import { LightningElement,wire,api } from 'lwc';
import { getRelatedListRecords } from 'lightning/uiRelatedListApi';
const COLUMNS = [
{ label: 'Name', fieldName: 'Name' },
{ label: 'Email', fieldName: 'Email' },
{ label: 'Phone', fieldName: 'Phone' }
];
export default class GetRelatedContacts extends LightningElement {
error;
records;
@api recordId;
columns = COLUMNS;
@wire( getRelatedListRecords, {
parentRecordId: '$recordId',
relatedListId: 'Contacts',
fields: [ 'Contact.Id', 'Contact.Name', 'Contact.Email' , 'Contact.Phone']
} )listInfo( { error, data } ) {
if ( data ) {
let recordList = [];
data.records.forEach( obj => {
let recordobj = {};
recordobj.Id = obj.fields.Id.value;
recordobj.Name = obj.fields.Name.value;
recordobj.Email = obj.fields.Email.value;
recordobj.Phone = obj.fields.Phone.value;
recordList.push( recordobj );
} );
this.records = recordList;
} else if (error) {
this.records = undefined;
}
}
}