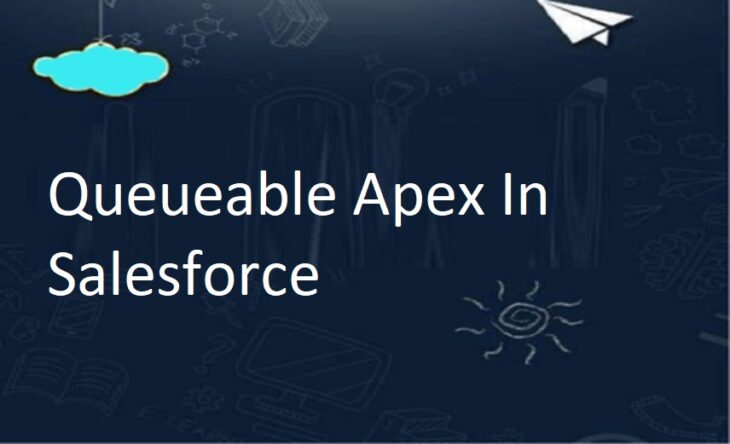
Queueable Apex Salesforce
Queueable Apex Salesforce is more advanced and enhanced version of future methods with some extra features.
By using the Queueable interface we can control asynchronous Apex processes. This interface enables you to add jobs to the queue and monitor them. Using the interface is an enhanced way of running your asynchronous Apex code compared to future methods. Queueable Apex class is an advanced version of the future methods.
Queueable Apex allows you to submit jobs for asynchronous processing similar to future methods with the following additional benefits:
- Chaining jobs: You can chain one job to another job by starting a second job from a running job. Chaining jobs is useful if you need to do some sequential processing.
- Monitoring: When you submit your job by invoking the
System.enqueueJob()
method, the method returns the ID of the AsyncApexJob record. You can use this ID to identify your job and monitor its progress, either through the Salesforce user interface in the Apex Jobs page, or programmatically by querying your record from AsyncApexJob. - Non-primitive types: Your
Queueable
class can contain member variables of non-primitive data types, such as sObjects or custom Apex types. Those objects can be accessed when the job executes. - You can add up to 50 jobs to the queue with System.enqueueJob in a single transaction.
Queueable Apex Class Syntax
public class AsyncExecutionExample implements Queueable {
public void execute(QueueableContext context) {
//your logic
}
}
Use Case:- A common scenario is to take some set of sObject records, execute some processing such as making a callout to an external REST endpoint or perform some calculations and then update them in the database asynchronously. Because @future
methods are limited to primitive data types (or arrays or collections of primitives), queueable Apex is an ideal choice.
The following code takes a collection of Account records, sets the parentId
for each record, and then updates the records in the database.
public class UpdateParentAccount implements Queueable {
private List<Account> accounts;
private ID parent;
public UpdateParentAccount(List<Account> records, ID id) {
this.accounts = records;
this.parent = id;
}
public void execute(QueueableContext context) {
for (Account account : accounts) {
account.parentId = parent;
// perform other processing or callout
}
update accounts;
}
}
For execute Queueable class, just open developer console and execute below code:-
// find all accounts in 'US'
List<Account> accounts = [select id from account where billingstate = 'US'];
// find a specific parent account for all records
Id parentId = [select id from account where name = 'ACME'][0].Id;
// instantiate a new instance of the Queueable class
UpdateParentAccount updateJob = new UpdateParentAccount(accounts, parentId);
// enqueue the job for processing
ID jobID = System.enqueueJob(updateJob);
New features of Spring 23 :-
Use the System.enqueueJob Method to Specify a Delay in Scheduling Queueable Jobs :-
A new optional override adds queueable jobs to the asynchronous execution queue with a specified minimum delay (0–10 minutes). Using the System.enqueue(queueable, delay)
method ignores any org-wide enqueue delay setting. The delay is ignored during Apex testing.
This example adds a job for delayed asynchronous execution by passing in an instance of your class implementation of the Queueable
interface for execution. There’s a minimum delay of 5 minutes before the job is executed.
Integer delayInMinutes = 5;
ID jobID = System.enqueueJob(new UpdateParentAccount(), delayInMinutes);